Why I Chose Sanity as the CMS for My Portfolio with Next.js 15
Created at 23/02/2025 by Negm
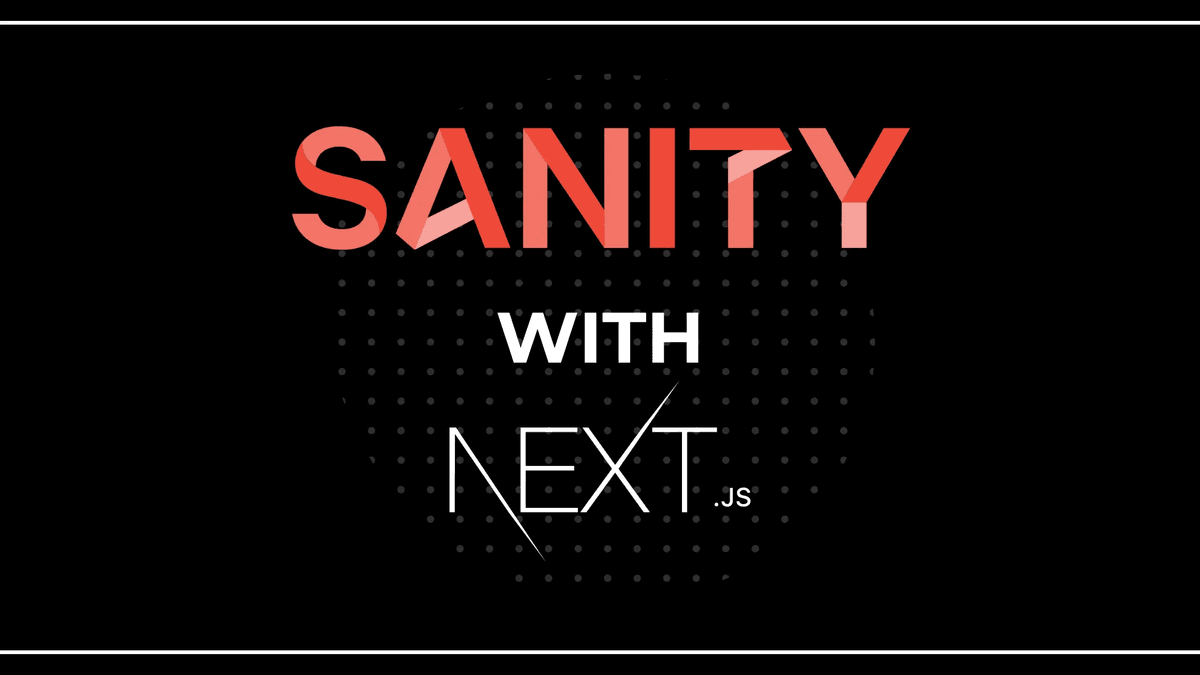
As a frontend developer, I wanted my portfolio to be dynamic, flexible, and easy to manage. After exploring different CMS options, I decided to go with Sanity as my headless CMS, paired with Next.js 15. This combination provided the perfect balance of customization, performance, and developer experience. In this blog post, I'll walk you through why I chose Sanity, how it integrates seamlessly with Next.js 15, and why it’s an excellent choice for developers looking to build a dynamic website.
Why Sanity?
1. Real-time Content Editing with GROQ
Sanity offers a real-time, collaborative editing experience that makes content management incredibly smooth. Unlike traditional CMS platforms, Sanity allows you to query data using GROQ, a flexible and powerful query language. This lets me fetch exactly the data I need without unnecessary overhead, keeping my portfolio lightweight and efficient.
2. Fully Customizable Content Structure
Sanity doesn’t force you into a predefined content structure. Instead, it gives you full control over how you model your content. This means I can structure my projects, blogs, and personal details exactly how I want, making it easy to scale and update in the future.
3. Headless CMS with API-first Approach
Since Sanity is headless, it provides a robust API that allows Next.js 15 to consume content effortlessly. I don’t have to deal with bulky admin panels or complex backend setups. Instead, I fetch the data through Sanity’s API, keeping my site highly performant and modular.
4. Great Developer Experience with Sanity Studio
Sanity Studio, the CMS dashboard, is built with React and fully customizable. This means I can tailor the interface to fit my exact needs, whether it’s adding custom input components or modifying the editor’s layout.
Why Sanity + Next.js 15 is a Perfect Match
1. Incremental Static Regeneration (ISR) for Performance
With Next.js 15, I can use Incremental Static Regeneration (ISR) to update my portfolio dynamically without rebuilding the entire site. Sanity’s real-time data updates fit perfectly into this workflow, ensuring that my portfolio remains fast and always up-to-date.
2. On-Demand Revalidation for Instant Updates
Sanity’s webhook system works seamlessly with Next.js’s on-demand revalidation, allowing me to instantly reflect content changes on my portfolio without waiting for a full deployment.
3. Optimized Image Handling with Sanity’s Image API
Sanity provides a powerful Image API that lets me optimize and transform images on the fly. Combined with Next.js’s built-in image component, this ensures that my portfolio loads quickly while maintaining high-quality visuals.
4. TypeScript Support for Type-Safe Content
Both Sanity and Next.js 15 offer first-class TypeScript support, making it easy to define and enforce content structures. This improves code maintainability and helps catch errors early in development.
Building & Deep Diving into Sanity + Next.js 15
1. Setting Up Sanity with Next.js
Getting started with Sanity and Next.js is straightforward. You can set up a Sanity CMS instance with:
1npx sanity@latest init
2
3
Then, install the Sanity client in your Next.js 15 project:
1npm install @sanity/client
2. Fetching Data from Sanity in Next.js
With GROQ, querying content is simple. Here’s an example of fetching blog posts:
1import { createClient } from '@sanity/client';
2
3const client = createClient({
4 projectId: 'your_project_id',
5 dataset: 'production',
6 useCdn: true,
7 apiVersion: '2024-02-23',
8});
9
10export async function getPosts() {
11 return await client.fetch(`*[_type == "post"]{title, slug, body}`);
12}
3. Rendering Content with Next.js 15
After fetching the content, you can render it in your Next.js pages like this:
1export default async function BlogPage() {
2 const posts = await getPosts();
3 return (
4 <div>
5 {posts.map((post) => (
6 <article key={post.slug}>
7 <h2>{post.title}</h2>
8 <p>{post.body}</p>
9 </article>
10 ))}
11 </div>
12 );
13}
Final Thoughts
Choosing Sanity as the CMS for my portfolio has been a game-changer. It gives me full control over content management while integrating seamlessly with Next.js 15 to deliver a fast, dynamic, and scalable website. Whether you're a solo developer building a personal site or a team working on a large-scale project, Sanity + Next.js 15 is a powerhouse combination worth considering.
Are you using Sanity in your projects?