How To Make This Cool Button in React.js with Tailwind CSS
Created at 03/02/2025 by Negm
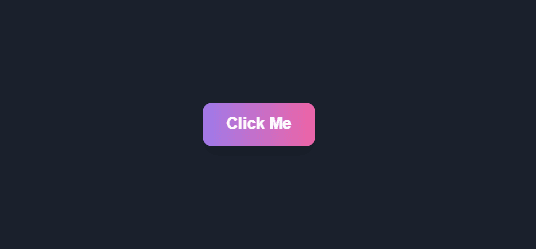
How To Make This Cool Button in React.js with Tailwind CSS
Buttons are essential UI elements, and adding stylish hover effects can enhance user experience. In this tutorial, we'll create a gradient hover effect button using React.js and Tailwind CSS.
Step 1: Setting Up the Project
Ensure you have a React project set up with Tailwind CSS. If you haven't installed Tailwind CSS yet, follow these steps:
1npx create-react-app gradient-button
2
3
4cd gradient-button
5
6
7npm install -D tailwindcss postcss autoprefixer
8
9
10npx tailwindcss init -p
Then, configure Tailwind by adding the following content to
1tailwind.config.js:
2
3module.exports = { content: ["./src/**/*.{js,jsx,ts,tsx}"],
4theme: {extend: {},},
5plugins: [],
6};
Include Tailwind in index.css
:
1@tailwind base;
2@tailwind components;
3@tailwind utilities;
Step 2: Creating the Gradient Button Component
Now, create a Button.js
component inside the src/components
folder.
1const GradientButton = ({ text }) => {
2return (
3<button className="relative px-6 py-3 font-bold text-white transition-all duration-300 rounded-lg bg-gradient-to-r from-purple-500 to-pink-500 hover:from-pink-500 hover:to-purple-500 shadow-lg hover:shadow-xl">
4{text}
5</button>);};
6
7export default GradientButton;
Step 3: Using the Button in Your App
Open App.js
and import the GradientButton
component.
1import GradientButton from "./components/Button";
2
3function App() {
4return (
5<div className="flex items-center justify-center min-h-screen bg gray-900">
6<GradientButton text="Click Me" />
7</div>
8);}
9
10export default App;
Step 4: Running the Application
Start your React app by running:
1npm start
Now, you have a stylish gradient button with a smooth hover effect! 🎨🔥
You can find a codepen full code at this link
Conclusion
With just a few lines of Tailwind CSS, we created a stunning gradient button with a hover transition. You can further customize colors and sizes to fit your design needs.
Happy coding! 🚀