Fetch vs Axios in React.js: Which One Should You Use?
Created at 08/02/2025 by Negm
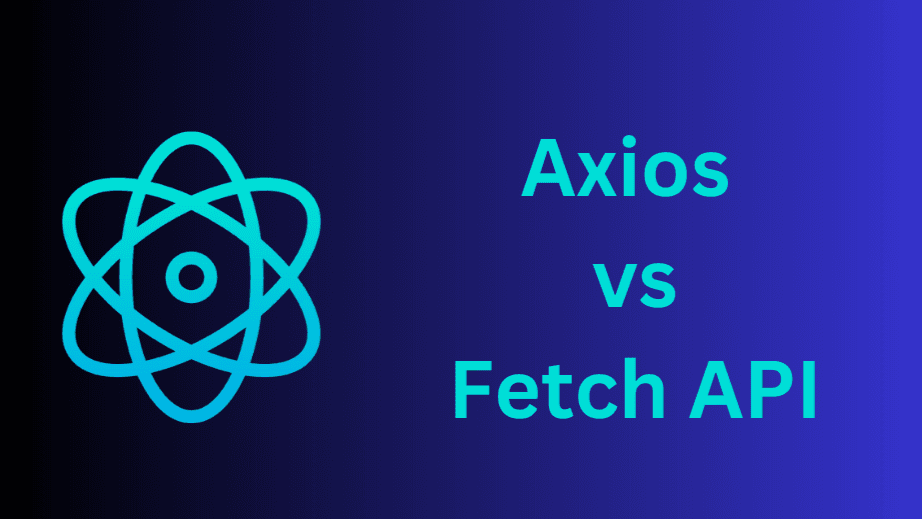
Fetch vs Axios in React.js: Which One Should You Use?
When working with APIs in React.js, two popular choices for making HTTP requests are Fetch API and Axios. Both have their strengths and weaknesses, so choosing the right one depends on your project needs. In this article, we’ll compare Fetch and Axios based on different factors such as ease of use, features, error handling, and performance.
1. What is Fetch API?
The Fetch API is a built-in JavaScript method for making HTTP requests. It is modern, promise-based, and provides a flexible way to interact with APIs.
Example of Fetch in React:
1useEffect(() => {
2fetch('https://jsonplaceholder.typicode.com/posts')
3.then(response => response.json())
4.then(data => console.log(data))
5.catch(error => console.error('Error:', error));
6}, []);
2. What is Axios?
Axios is a third-party library that simplifies HTTP requests. It is promise-based like Fetch but provides additional features such as request cancellation, automatic JSON parsing, and response timeouts.
Example of Axios in React:
1import axios from 'axios';
2
3useEffect(() => {
4axios.get('https://jsonplaceholder.typicode.com/posts')
5.then(response => console.log(response.data))
6.catch(error => console.error('Error:', error));
7}, []);
3. Key Differences Between Fetch and Axios
Fetch :
Built-in: Yes (native JS API)
Automatic JSON Parsing: No (must use .json()
)
Error Handling: Only rejects on network failures
Request Timeout: No built-in support
Request Cancellation: No built-in support
Interceptors: No
Browser Compatibility: Modern browsers
Axios :
Built-in: No (requires installation)
Automatic JSON Parsing: Yes (response data is already parsed)
Error Handling: Rejects on HTTP errors & network failures
Request Timeout: Yes, supports timeouts
Request Cancellation: Yes, using CancelToken
Interceptors: Yes, allows modifying requests/responses
Browser Compatibility: Works in all browsers
4. Error Handling
Handling errors is easier with Axios because it automatically rejects the promise for non-2xx HTTP status codes.
1fetch('https://jsonplaceholder.typicode.com/posts')
2.then(response => {
3if (!response.ok) {
4throw new Error('Network response was not ok');
5}
6return response.json();
7})
8.catch(error => console.error('Fetch Error:', error));
Axios Error Handling:
1axios.get('https://jsonplaceholder.typicode.com/posts')
2.then(response => console.log(response.data))
3.catch(error => console.error('Axios Error:', error));
5. When to Use Fetch or Axios?
- Use Fetch if you want a lightweight, native solution and don’t need advanced features like request cancellation or interceptors.
- Use Axios if you need automatic JSON parsing, better error handling, request timeouts, or want a more developer-friendly experience.
Conclusion
Both Fetch and Axios are great options for making HTTP requests in React. Fetch is a good choice for simple requests, while Axios is better suited for more complex scenarios where you need additional features. If your project requires better error handling and configuration options, Axios is the way to go. Otherwise, Fetch works just fine for most basic API calls.
Which one do you prefer? 🚀